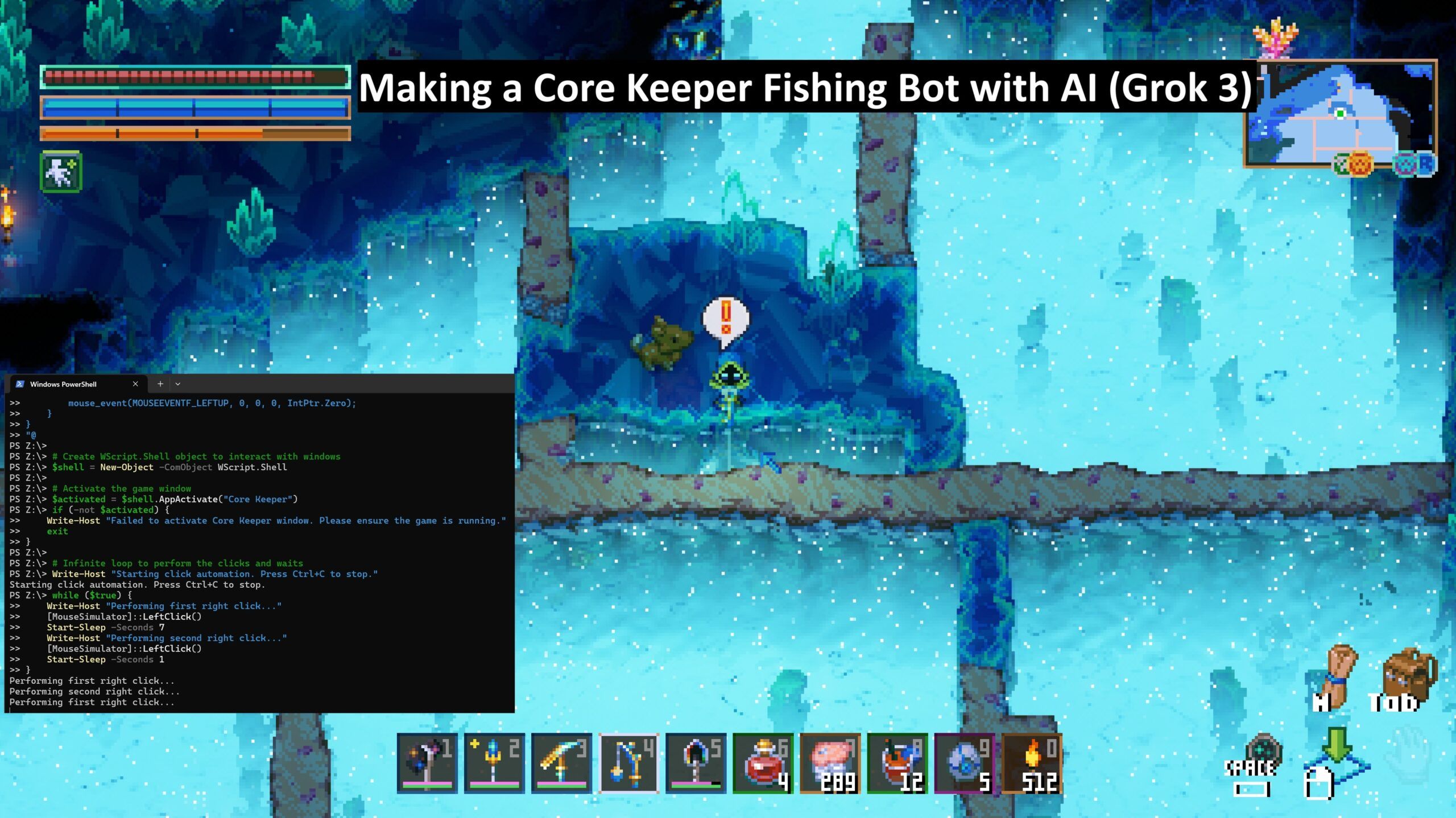
Despite the massive hype around AI I have always had a lot of trouble getting the AI to generate usable code. Even when I reply back and try to help the AI understand what it’s missing it often tends to actually get *worse* after my suggestions.
Even for this simple project ChatGPT and Gemini failed miserably to write a very simple fishing bot. They both hallucinated and tried using PowerShell modules that don’t exist. Trying to further refine my prompts only yielded *worse* results!
After hearing all the hype around Grok 3 I decided to give it a try at writing something very simple I wanted: something to simply click the mouse for me in Core Keeper in order to fish. To my surprise in “Think” mode Grok 3 was able to give me incredibly simple and effective code in one try. Wow!
My Prompt
My prompt to Grok 3 was simply this:
Write a PowerShell script that sends keystrokes to the game “Core Keeper” simply right clicking and waiting 8 seconds then right clicking again. After that it should recast one second later and then wait another 8 seconds
The winning prompt for Grok 3 in “Think” mode to write a Core Keeper fishing bot successfully
Why did I choose PowerShell? Because it’s built into the Windows operating system natively. You don’t have to download anything. You just launch PowerShell from the start menu and paste the code.
Using the Bot
Here is the PowerShell code that Grok 3 produced:
# Define the MouseSimulator class to handle mouse events
Add-Type -TypeDefinition @"
using System;
using System.Runtime.InteropServices;
public class MouseSimulator {
[DllImport("user32.dll", SetLastError = true)]
public static extern void mouse_event(uint dwFlags, uint dx, uint dy, uint dwData, IntPtr dwExtraInfo);
public const uint MOUSEEVENTF_RIGHTDOWN = 0x0008;
public const uint MOUSEEVENTF_RIGHTUP = 0x0010;
public static void RightClick() {
mouse_event(MOUSEEVENTF_RIGHTDOWN, 0, 0, 0, IntPtr.Zero);
mouse_event(MOUSEEVENTF_RIGHTUP, 0, 0, 0, IntPtr.Zero);
}
}
"@
# Create WScript.Shell object to interact with windows
$shell = New-Object -ComObject WScript.Shell
# Activate the game window
$activated = $shell.AppActivate("Core Keeper")
if (-not $activated) {
Write-Host "Failed to activate Core Keeper window. Please ensure the game is running."
exit
}
# Infinite loop to perform the clicks and waits
Write-Host "Starting click automation. Press Ctrl+C to stop."
while ($true) {
Write-Host "Performing first right click..."
[MouseSimulator]::RightClick()
Start-Sleep -Seconds 8
Write-Host "Performing second right click..."
[MouseSimulator]::RightClick()
Start-Sleep -Seconds 1
}
The way you use the bot is to move your character to the spot in Core Keeper that you want to fish at and put your mouse cursor over the tile you will be fishing.
Now minimize the game and open the start menu and type “PowerShell”. You should see Windows PowerShell come up as an option to open up. Open PowerShell and simply paste the code.
By default the code waits 8 second and then reels in. You may need to adjust this depending on your fishing skill. When I was getting close to 100 fishing I had to lower the sleep timer down to 7 and even closer to 6 seconds. This is done by adjusting the line that says “Start-Sleep -Seconds 8”. Simply change this to the number of seconds that is appropriate for your fishing power.
Once you have it tuned it won’t get 100% of all of the fish but it will get most of them. It got me to level 100 fishing fairly quickly.
Gemini’s Failed Attempts
I have access to the “Pro” version of Gemini due to my Pixel 9 Pro Fold coming with 1 year of it. My prompt to Gemini was:
Write a simple fishing bot in Windows PowerShell that sends a right click to the window titled “Core Keeper” and waits 6 seconds then right clicks to reel in the fish, then about 1 second later casts again to wait another 6 seconds and repeat
Pretty dang similar to what I gave to Grok 3. What was the result? This:
# Requires the 'SendInput' module. Install if needed:
# Install-Module -Name SendInput
Add-Type -AssemblyName System.Windows.Forms
# Function to send a right-click to a specific window
function Send-RightClickToWindow {
param(
[string]$WindowTitle
)
# Find the window by title
$window = Get-Process | Where-Object {$_.MainWindowTitle -like "*$WindowTitle*"}
if ($window) {
# Bring the window to the foreground (activate it)
[System.Windows.Forms.Application]::Activate($window.MainWindowHandle)
Start-Sleep -Milliseconds 100 # Short pause to let the window activate
# Send a right-click using SendInput module (more reliable than mouse_event)
[WindowsInput.InputSimulator]::new().Mouse.RightButtonClick()
Write-Host "Right-click sent to '$WindowTitle'"
} else {
Write-Warning "Window '$WindowTitle' not found."
}
}
# Main fishing loop
while ($true) {
# Cast (Right-click)
Send-RightClickToWindow -WindowTitle "Core Keeper"
# Wait for the fish to bite (adjust timing as needed)
Write-Host "Waiting for bite (6 seconds)..."
Start-Sleep -Seconds 6
# Reel in (Right-click)
Send-RightClickToWindow -WindowTitle "Core Keeper"
# Wait before casting again (adjust timing as needed)
Write-Host "Reeling and preparing to cast again (1 second)..."
Start-Sleep -Seconds 1
}
Write-Host "Fishing bot stopped." # This line will likely never be reached in the infinite loop.
Oh boy. Right off the bat we’re trying to install a PowerShell module called SendInput? What on earth is that? Does it even exist?

It sure doesn’t! There is no such thing. It’s not real. This is a hallucination. It’s not the only mistake Gemini made either. There’s also this line:
[System.Windows.Forms.Application]::Activate($window.MainWindowHandle)
That looks pretty reasonable at first glance. The problem? There is no such static method available. This is *also* a hallucination.
No matter how many times I responded back to Gemini (which recognized that the method didn’t exist) it kept giving me code that tried to utilize this invalid method. That’s a fail.
ChatGPT’s Failed Attempts
So how about ChatGPT? Here was my prompt:
Write a simple fishing bot in Windows PowerShell that sends a right click to the window titled “Core Keeper” and waits 6 seconds then right clicks to reel in the fish
Here is what I got:
Add-Type @"
using System;
using System.Runtime.InteropServices;
public class WinAPI {
[DllImport("user32.dll", SetLastError = true)]
public static extern IntPtr FindWindow(string lpClassName, string lpWindowName);
[DllImport("user32.dll", SetLastError = true)]
public static extern IntPtr PostMessage(IntPtr hWnd, uint Msg, IntPtr wParam, IntPtr lParam);
}
"@
$WM_RBUTTONDOWN = 0x0204
$WM_RBUTTONUP = 0x0205
# Find the Core Keeper window
$gameWindow = [WinAPI]::FindWindow($null, "Core Keeper")
if ($gameWindow -eq [IntPtr]::Zero) {
Write-Host "Core Keeper window not found!"
exit
}
function Send-RightClick {
param($hWnd)
# Simulate right mouse button down
[WinAPI]::PostMessage($hWnd, $WM_RBUTTONDOWN, [IntPtr]::Zero, [IntPtr]::Zero)
Start-Sleep -Milliseconds 100
# Simulate right mouse button up
[WinAPI]::PostMessage($hWnd, $WM_RBUTTONUP, [IntPtr]::Zero, [IntPtr]::Zero)
}
while ($true) {
Write-Host "Casting fishing rod..."
Send-RightClick -hWnd $gameWindow
Start-Sleep -Seconds 6
Write-Host "Reeling in..."
Send-RightClick -hWnd $gameWindow
Start-Sleep -Seconds 1
}
Again, like Gemini that looks pretty reasonable but the code has several problems. The FindWindow method it’s using never finds the window.
I asked ChatGPT to try using an alternative method to find the window and it decided to use Get-Process instead to find the process ID. It spit out a more complicated version that could not find the window either.
How About Deepseek R1?
Oh I just knew you were going to ask. Interestingly DeepSeek R1 generated almost the exact same code as ChatGPT:
Add-Type -TypeDefinition @"
using System;
using System.Runtime.InteropServices;
public class User32 {
[DllImport("user32.dll")]
public static extern IntPtr FindWindow(string lpClassName, string lpWindowName);
[DllImport("user32.dll")]
public static extern bool SetForegroundWindow(IntPtr hWnd);
[DllImport("user32.dll")]
public static extern int SendMessage(IntPtr hWnd, uint Msg, int wParam, int lParam);
}
"@
# Replace "Core Keeper" with the exact window title of the game
$windowTitle = "Core Keeper"
$hWnd = [User32]::FindWindow($null, $windowTitle)
if ($hWnd -eq [IntPtr]::Zero) {
Write-Host "Window '$windowTitle' not found!"
exit
}
# Bring the game window to the foreground
[User32]::SetForegroundWindow($hWnd) | Out-Null
# Simulate right-click (mouse button down and up)
function Simulate-RightClick {
[User32]::SendMessage($hWnd, 0x0204, 0x0002, 0) # Right mouse button down
Start-Sleep -Milliseconds 50
[User32]::SendMessage($hWnd, 0x0205, 0x0002, 0) # Right mouse button up
}
# Loop to perform the actions
while ($true) {
# First right-click
Simulate-RightClick
Start-Sleep -Seconds 8
# Second right-click
Simulate-RightClick
Start-Sleep -Seconds 1
# Recast (right-click again)
Simulate-RightClick
Start-Sleep -Seconds 8
}
This bot suffers from the exact same issues as the ChatGPT one. The problem is identical. That FindWindow approach it is taking does not work properly.
Interestingly DeepSeek R1 made a mistake that no other AI made. If you look at the loop it put my recast inside the main loop:
while ($true) {
# First right-click
Simulate-RightClick
Start-Sleep -Seconds 8
# Second right-click
Simulate-RightClick
Start-Sleep -Seconds 1
# Recast (right-click again)
Simulate-RightClick
Start-Sleep -Seconds 8
}
That’s actually 3 actions. Both other AIs understood that I wanted it to cast once and then reel it in and repeat that sequence over and over. DeepSeek R1 instead made it cast, reel in, and then cast again and then it restarts the loop (which is the wrong behavior).
So, hail China? Not yet. To my surprise DeepSeek R1 actually did the worst on this task despite being a deep thinking model.
Conclusion
Grok 3 absolutely nailed it. It is what it is. It’s the only one that could that I tried.
ChatGPT and Gemini could not get it right no matter how many prompts I gave them. DeepSeek R1 not only made the same mistake as ChatGPT but made additional ones in interpreting what I was asking for (such as casting *twice* in the same loop instead of casting once and then reeling in like it should).
I want to see more of whatever Grok is doing so that it’s not straight up making things up in a coding context (such as libraries and modules that don’t even exist). The hallucinations are completely inappropriate for coding. ChatGPT and Gemini still suffer *heavily* from these hallucinations (even in a coding context).
Was it necessary to get an AI to write this script for me? No. I’ve been scripting / coding for a long time and could have easily wrote this on my own. The point of the exercise though was to evaluate whether these tools are actually getting good enough to reliably write basic scripts / code without hallucinating.
The answer? They’re getting a lot closer and Grok 3 seems to be leading the pack right now!